Identify Which Page is this in Magento 2
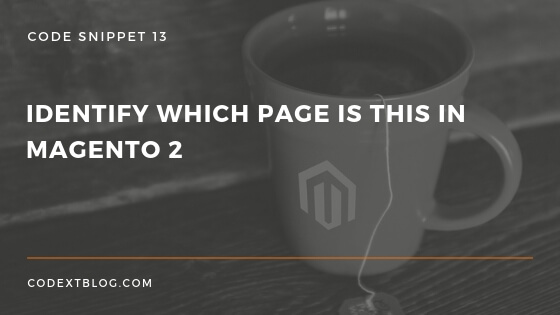
As a Magento 2 developer many times you want to load the content based on the page types. Like you want to populate the static block on certain pages only or want to check current page is a home page, category page, product page & cms page in Magento 2. Therefore you first need to identify which page is this in Magento 2 using PHP code.
In this code snippet, we will create a helper file and define some functions. These functions will identify which page is this in Magento 2. After that, we will create a phtml file and see how we can call a helper function to identify page type in the phtml file.
Create Helper File
Create Data.php file under app/code/Vendor/Module/Helper
directory and add below code.
<?php namespace Vendor\Module\Helper; class Data extends \Magento\Framework\App\Helper\AbstractHelper { public function isHomePage() { if($this->_request->getFullActionName() == 'cms_index_index') return true; } public function isCategoryPage() { if($this->_request->getFullActionName() == 'catalog_category_view') return true; } public function isProductPage() { if($this->_request->getFullActionName() == 'catalog_product_view') return true; } public function isCartPage() { if($this->_request->getFullActionName() == 'checkout_cart_index') return true; } public function isCheckoutPage() { if($this->_request->getFullActionName() == 'checkout_index_index') return true; } public function isCmsPage() { if($this->_request->getFullActionName() == 'cms_page_view') return true; } }
Create PHTML File
Create test.phtml file under app/code/Vendor/Module/view/frontend/templates
directory and add below code.
<?php $helper = $this->helper('Vendor\Module\Helper\Data'); if($helper->isHomePage()) { //your code here } if($helper->isCategoryPage()) { //your code here } if($helper->isProductPage()) { //your code here } if($helper->isCartPage()) { //your code here } if($helper->isCheckoutPage()) { //your code here } if($helper->isCmsPage()) { //your code here }
Hope this code snippet will help you while developing your Magento 2 application. Consider like our page on Facebook and follow us on Twitter if you find this useful.
Leave a Comment
(0 Comments)
Useful Magento 2 Articles
Author Info
Chirag
Connect With Me