Get Product Information From Product Id in Magento 2
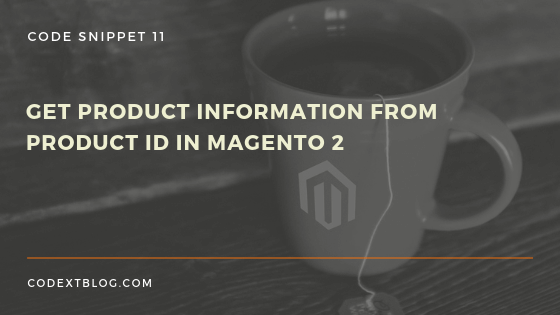
Many times in your Magento 2 projects you need to get product information from product id. There are several ways you can get product information from product id. In this post, we will see different methods to get product information from product id in Magento 2.
Using Construct Or Dependency Injection Method
This is the most used and recommended method by Magento 2 Dev Doc.
To get the product information you first have to load the particular product object. There are two methods available to load product. One is using Factory Pattern and another is using Repository Pattern. Let’s see code snippet of both methods.
1. Using Factory Pattern
namespace Codextblog\Pcollection\Block\Index; class Index extends \Magento\Framework\View\Element\Template { protected $_productFactory; public function __construct( \Magento\Backend\Block\Template\Context $context, \Magento\Catalog\Model\ProductFactory $productFactory, array $data = [] ) { $this->_productFactory = $productFactory; parent::__construct($context, $data); } public function getProductInformation() { $productId = 10; $product = this->_productFactory->create()->load($productId); //get all information echo "<pre>"; print_r($product->getData()); //get specific information echo $product->getName() . '<br>'; echo $product->getSKU() . '<br>'; } }
2. Using Repository Pattern
namespace Codextblog\Pcollection\Block\Index; class Index extends \Magento\Framework\View\Element\Template { protected $_productRepository; public function __construct( \Magento\Backend\Block\Template\Context $context, \Magento\Catalog\Model\ProductRepository $productRepository, array $data = [] ) { $this->_productFactory = $productFactory; parent::__construct($context, $data); } public function getProductInformation() { $productId = 10; $product = $this->_productRepository->getById($productId); //get all information echo "<pre>"; print_r($product->getData()); //get specific information echo $product->getName() . '<br>'; echo $product->getSKU() . '<br>'; } }
Using ObjectManager (Not Recommended)
You can get product information from product id using object manager in any file of your Magento 2 project. But this is not the recommended method. One should always use any of the above two methods.
<?php $objectManager = \Magento\Framework\App\ObjectManager::getInstance(); $productRepository = $objectManager->get('\Magento\Catalog\Model\ProductRepository'); $productId = 10; $product = $productRepository->getById($productId); //get all information echo "<pre>"; print_r($product->getData()); //get specific information echo $product->getName() . '<br>'; echo $product->getSKU() . '<br>';
That’s it. Today we have indentified all the available methods magento 2 offers to get product information from product id. To receive update about this type of code snippet, please like us on Facebook and follow us on Twitter.
Leave a Comment
(0 Comments)
Useful Magento 2 Articles
Author Info
Chirag
Connect With Me