How to Read CSV Data as an Array in Magento 2
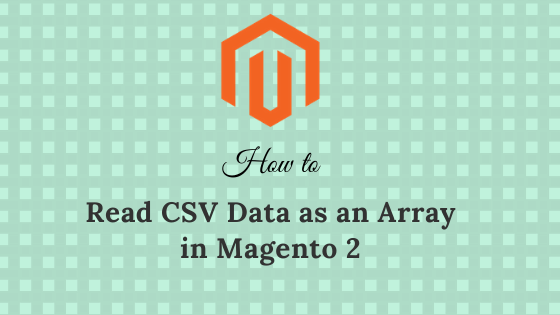
Sometime as a Magento 2 developer you want to fetch the third party data in a form of CSV file. However you do not need all data from it. At that time if you convert the CSV data in to array then it becomes very easy to manipulate. Using Magento\Framework\File\Csv
built in class, you can read csv data as an array in Magento 2.
Read CSV Data as an Array in Magento 2
<?php namespace Codextblog\Demo\Helper; use Magento\Framework\App\Helper\AbstractHelper; use Magento\Framework\File\Csv; use Magento\Framework\Filesystem\Driver\File; use Magento\Framework\App\Filesystem\DirectoryList; use Magento\Framework\Exception\FileSystemException; class Price extends AbstractHelper { /** * @var DirectoryList */ protected $directoryList; /** * @var Csv */ protected $csv; /** * @var File */ protected $file; public function __construct( Context $context, DirectoryList $directoryList, Csv $csv, File $file ) { parent::__construct($context); $this->directoryList = $directoryList; $this->csv = $csv; $this->file = $file; } public function readCsv($csvFilePath) { $rootDirectory = $this->directoryList->getRoot(); $csvFile = $rootDirectory . "/" . $csvFilePath; try { if ($this->file->isExists($csvFile)) { //set delimiter, for tab pass "\t" $this->csv->setDelimiter(","); //get data as an array $data = $this->csv->getData($csvFile); if (!empty($data)) { // ignore first header column and read data foreach (array_slice($data, 1) as $key => $value) { $columnFirst = trim($value['0']); $columnSecond = trim($value['1']); //and so on. } } } else { $this->_logger->info('Csv file not exist'); return __('Csv file not exist'); } } catch (FileSystemException $e) { $this->_logger->info($e->getMessage()); } } }
In line number 48 we are using getData
function to read csv data as an array. There is one more method called getDataPairs
that read csv data as a pair array. That means array key is your first column and value is your rest of the column.
I hope this post will be helpful to you in your project. Correction or improvements to this code is highly appreciateble.
Leave a Comment
(1 Comment)
Hi Chirag,
How to Get first row like (header) from Csv file without foreach loop ?
Thanks
Useful Magento 2 Articles
Author Info
Chirag
Connect With Me