Programmatically Create Invoice in Magento 2 module [updated]
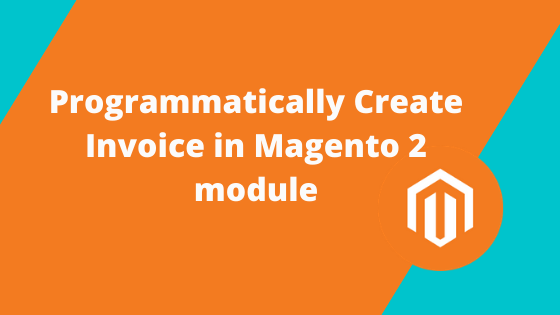
Magento 2 order management system creates an invoice when an order is paid online. If you are using PayPal, authorize.net, stripe, etc payment methods, it will create an invoice upon the capture of the amount. However, when we are using offline payment methods, we have to create invoices in Magento 2 manually.
In that case, we have two options. The first option is creating an invoice from the backend order page and the second option is programmatically to create an invoice in Magento 2 module.
Therefore, Today we will see how to programmatically create invoices in Magento 2 module.
Programmatically Create Invoice in Magento 2
<?php namespace Codextblog\Demo\Cron; use Magento\Sales\Api\OrderRepositoryInterface; use Magento\Sales\Model\Service\InvoiceService; use Magento\Framework\DB\TransactionFactory; use Codextblog\Demo\Logger\Logger; class Invoicecreation { /** * @var OrderRepositoryInterface */ protected $orderRepository; /** * @var InvoiceService */ protected $invoiceService; /** * @var TransactionFactory */ protected $transactionFactory; /** * @var Logger */ protected $customLogger; public function __construct( OrderRepositoryInterface $orderRepository, InvoiceService $invoiceService, TransactionFactory $transactionFactory, Logger $customLogger ) { $this->orderRepository = $orderRepository; $this->invoiceService = $invoiceService; $this->transactionFactory = $transactionFactory; $this->customLogger = $customLogger; } public function execute() { $orderId = 111; $order = $this->orderRepository->get($orderId); if ($order->canInvoice()) { $invoice = $this->invoiceService->prepareInvoice($order); $invoice->setRequestedCaptureCase(Invoice::CAPTURE_ONLINE); $invoice->register(); $invoice->getOrder()->setCustomerNoteNotify(false); $invoice->getOrder()->setIsInProcess(true); $order->addCommentToStatusHistory(__('Automatically INVOICED'), false); $transactionSave = $this->transactionFactory->create(); $transactionSave->addObject($invoice)->addObject($invoice->getOrder()); $transactionSave->save(); $this->customLogger->info('Invoice created for order '.$orderId); } else { $this->customLogger->info('Invoice can not be created for order '.$orderId); } } }
In line 55, we have set customer notification to false. That means invoice email will be not sent to the customer and the customer will not get an email notification. However, you can turn it on by setting it to true.
In conclusion, programmatically create invoice in Magento 2 is the best automation way for offline payment methods.
Leave a Comment
(0 Comments)
Useful Magento 2 Articles
Author Info
Chirag
Connect With Me